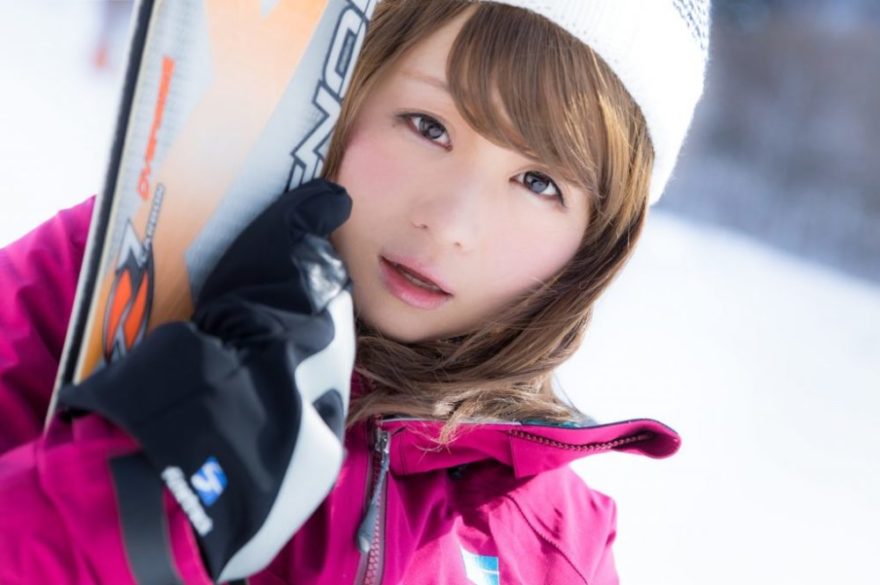
Laravel(ララベル)で開発してて、いつもコマンド忘れてるので
メモ…
テーブルを追加する
一応今回の対象はこんな環境です。
開発環境
OS: CentOS7.x PHP: 7.2.12 Laravel: 5.7
テーブル作成のマイグレーションを行う
作りたいテーブルの構成
作りたいテーブルの構成をこんな感じとしましょう。
テーブル名: members
カラム名 | 型とか |
---|---|
id | ID auto_increment |
login_id | ログインID、一意、varchar(64) |
name | お名前、varchar(128) |
password | パスワード |
テーブル作成用マイグレーションファイルの生成
このとき、このようなコマンドでまずマイグレーションファイルを作ります。 php artisan make:migration create_members_table
コマンド発行後、こんなメッセージが返ってきます。 Created Migration: 2018_xx_xx_xxxxxx_create_members_table
すると、Laravelプロジェクトのディレクトリの
database/migiration/ 以下に
2018_xx_xx_xxxxxx_create_members_table.php
が生成されていますのでそれをダウンロードします。
内容はこんな感じ。
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateMembersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('members', function (Blueprint $table) { $table->increments('id'); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('members'); } }
そこにテーブル構成に沿って他の要素を組み込んでやります。
<?php use Illuminate\Support\Facades\Schema; use Illuminate\Database\Schema\Blueprint; use Illuminate\Database\Migrations\Migration; class CreateMembersTable extends Migration { /** * Run the migrations. * * @return void */ public function up() { Schema::create('members', function (Blueprint $table) { $table->increments('id'); $table->string("login_id", 64)->unique(); $table->string("name", 128); $table->string("password"); $table->timestamps(); }); } /** * Reverse the migrations. * * @return void */ public function down() { Schema::dropIfExists('members'); } }
文字色が「赤」になっているところが追記した箇所です。
それをアップロードしてあげます。(同じ場所に上書きします)
作成処理実行
その後、下記コマンドを発行します。
php artisan migrate
コマンド発行後、こんな感じに返ってくれば成功です。
Migrating: 2018_xx_xx_xxxxxx_create_members_table
Migrated: 2018_xx_xx_xxxxxx_create_members_table
実際にデータベースを見ると出来上がってます。
指定したカラム以外に勝手に自動的に下記カラムも作ってくれます。
カラム名 | 初期値 |
---|---|
created_at | timestamp NULL |
updated_at | timestamp NULL |
あとはモデルとか作って・・
このテーブルをLaravelで操作するためにモデルを作ります。 php artisan make:model Member
このコマンドを発行します!
Model created successfully.
メッセージが返ってきて成功です。
作られたモノは app/ にあります。
app/Member.php
中身はこんな感じ。
<?php namespace App; use Illuminate\Database\Eloquent\Model; class Member extends Model { // }
ここからこのモデルを 1:1 にしたい とか 1:多 にしたいとかいろいろ出来ます。
Laravel便利!
とりあえず、こんな感じでw
参考資料
https://readouble.com/laravel/5.7/ja/migrations.html
https://readouble.com/laravel/5.7/ja/eloquent-relationships.html#one-to-many